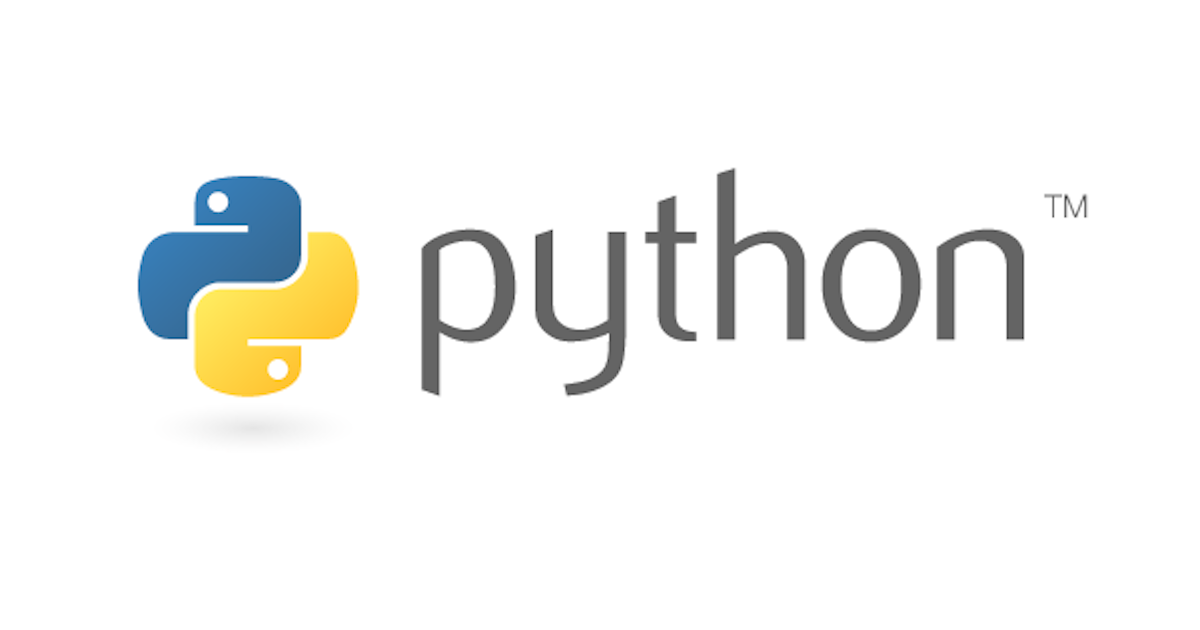
pythonのstatisticsを使って数理統計入門
pythonの標準ライブラリ「statistics」を使うと簡単に平均値、中央値、分散、標準偏差を求められます。
pythonでは標準ライブラリでstatistics - 数理統計関数が用意されています。
これを使えば、簡単に平均値、中央値、分散、標準偏差を求められます。
動作確認環境
$ python --version Python 3.8.5
平均値
算術平均
import statistics data = [13, 15, 25, 37, 40, 50, 57, 61, 90, 99] result = statistics.mean(data) print(result) >>> 48.7 1.30 ms
調和平均
import statistics data = [13, 15, 25, 37, 40, 50, 57, 61, 90, 99] result = statistics.harmonic_mean(data) print(result) >>> 32.178532252935725 1.17 ms
中央値
- median
- median_low
- median_high
中央値を算出する関数は3つありますが、リストの要素数が奇数の場合はどれも同じ結果になります。
import statistics data = [13, 15, 25, 37, 40, 50, 57, 61, 90, 99] result = statistics.median(data) print(result) >>> 45.0 1.10 ms
median関数でリストの要素数が偶数だった場合、中央の2値の平均を出します。
中央の2値の小さい方を返す
import statistics data = [13, 15, 25, 37, 40, 50, 57, 61, 90, 99] result = statistics.median_low(data) print(result) >>> 40 0.81 ms
中央の2値の大きい方を返す
import statistics data = [13, 15, 25, 37, 40, 50, 57, 61, 90, 99] result = statistics.median_high(data) print(result) >>> 50 1.01 ms
最頻値
データの中で最も頻度が高い値(モード)を返します。
同じ頻度の値が複数見つかった場合は最初に見つかった値を返します。
最頻値が見つからなかった場合は最初のインデックスの値を返します。
3.8より前のバージョンで複数の最頻値が見つかった場合は`StatisticsError`になりますのでご注意ください。
import statistics data = [13, 15, 25, 37, 40, 50, 57, 61, 90, 90] result = statistics.mode(data) print(result) >>> 90 1.04 ms
文字列もOK
statistics.mode(["red", "blue", "blue", "red", "green", "red", "red"]) >>> 'red'
分散と標準偏差
分散とはデータの散らばり具合を表す指標です。分散の正の平方根が標準偏差となります。
母標準偏差
import statistics data = [1.5, 2.5, 2.5, 2.75, 3.25, 4.75] result = statistics.pstdev(data) print(result) >>> 0.986893273527251 1.05 ms
母分散
import statistics data = [0.0, 0.25, 0.25, 1.25, 1.5, 1.75, 2.75, 3.25] result = statistics.pvariance(data) print(result) >>> 1.25 1.12 ms
標本標準偏差
import statistics data = [1.5, 2.5, 2.5, 2.75, 3.25, 4.75] result = statistics.stdev(data) print(result) >>> 1.0810874155219827 1.40 ms
標本標準分散
import statistics data = [2.75, 1.75, 1.25, 0.25, 0.5, 1.25, 3.5] result = statistics.variance(data) print(result) >>> 1.3720238095238095 1.33 ms